728x90
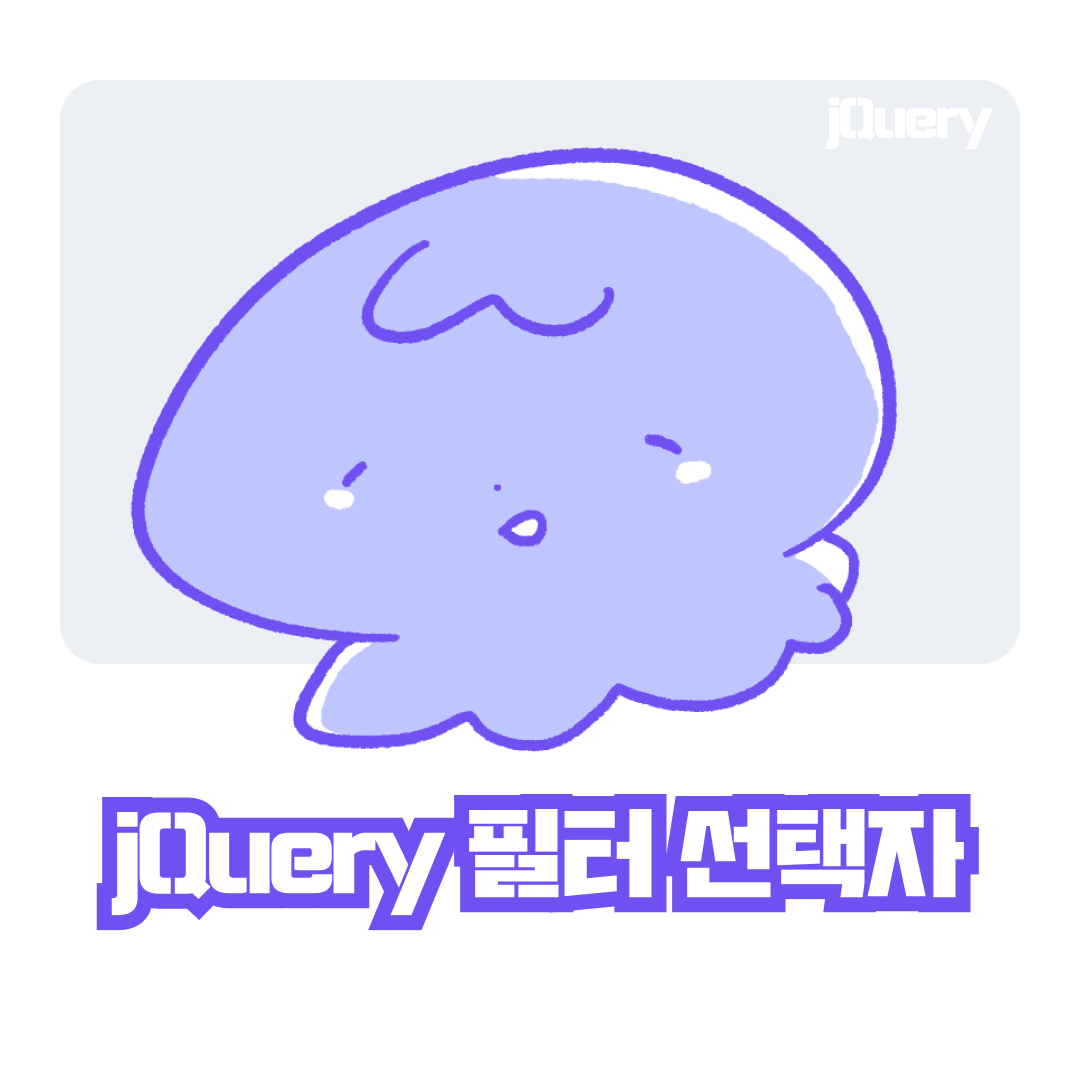
jQuery 필터 선택자
선택자 종류 | 설명 | |
---|---|---|
:even | $("tr:even") | tr요소 중 짝수 행만 선택합니다. |
:odd | $("tr:odd") | tr요소 중 홀수 행만 선택합니다. |
:first | $("td:first") | 첫번째 td요소를 선택합니다. |
:last | $("td:last") | 마지막 td요소를 선택합니다. |
:header | $(":header") | 헤딩 (h1~h6) 요소를 선택합니다. |
:eq() | $("li:eq(0)" | index가 0인 li요소를 선택합니다. index는 0번이 첫 번째 요소입니다. |
:gt() | $("li:gt()") | index가 0보다 큰 li요소들을 선택합니다. |
:lt() | $("li:lt(2)") | index가 2보다 작은 li요소들을 선택합니다. |
:not() | $("li:not(.bg)") | li요소 중에서 class명 bg가 아닌 li요소를 선택합니다. |
:root | $(".root") | html을 의미합니다. |
:animated | $(".animated") | 움직이는 요소를 선택합니다. |
위의 예시를 사용하여 jQuery를 사용해보았습니다.
♠ jQuery 필터 선택자의 코드만 보기
$(document).ready(function(){
$("tr:even").css("background","red")
$("tr:odd").css("background","orange")
$("td:first").css("background","yellow")
$("td:last").css("background","green")
$(":header").css("background","blue")
$("li:eq(0)").css("background","navy")
$("li:gt(0)").css("background","purple")
$("li:lt(3)").css("border", "4px solid gray")
$(":root").css("background", "lightgray")
(function upDown(){
$("h2").slideToggle(2000,upDown);
})();
$("animated").css("border","4px soild darkred")
})
◆ jQuery 자식 필터 선택자
jQuery에서 자식 필터 선택자 중 'child'가 붙은 선택자는 요소가 순차적으로 나열 되어 있을 때 사용하고, 'of-type'이 붙은 선택자는 요소가 순차적으로 나열되어 있지 않아도 동일 요소 이면 선택이 가능하다는 점을 이해해야 합니다.
선택자 종류 | 설명 | |
---|---|---|
:first-child | $("span:first-child") | 첫번째 span 요소를 선택합니다. |
:last-child | $("span:last-child") | 마지막 span 요소를 선택합니다. |
:first-of-type | $("span:first-of-type") | span 요소 중에서 첫번째 span 요소를 선택합니다. |
:last-of-type | $("span:last-of-type") | span 요소 중에서 마지막 span 요소를 선택합니다. |
:nth-child | $("span:nth-child(2)") |
두번째 span 요소를 선택합니다. nth-child(2n)은 2,4,6,...번째 요소를 선택하고, nth-child(2n+1)은 1,3,5,...번째 요소를 선택합니다. |
:nth-last-child | $("span:nth-last-child(2)") | 마지막에서 두 번째 span 요소를 선택합니다. |
:nth-of-type | $("span:nth-of-type(2)") | span 요소 중에서 두번째 span 요소를 선택합니다. |
:nth-last-of-type | $("span:nth-last-of-type(2)") | span 요소 중에서 마자막에서 두번째 span 요소를 선택합니다. |
:only-child | $("div > span:only-child") | div의 자식 요소에서 오직 span 요소가 하나만 있는 span 요소를 선택합니다. |
:only-of-type | $("div > span:only-of-type") | div의 자식 요소에서 span 요소가 하나만 있는 span 요소를 선택합니다. |
♠ jQuery 필터 선택자의 코드만 보기
$(document).ready(function() {
$("#m1 > span:first-child").css("border","2px solid red");
$("#m1 > span:last-child").css("border","2px solid red");
$("#m2 > span:first-of-type").css("border","2px solid orange");
$("#m2 > span:last-of-type").css("border","2px solid orange");
$("#m3 > span:nth-child(1)").css("border","2px solid yellow");
$("#m3 > span:nth-last-child(1)").css("border","2px solid yellow");
$("#m4 > span:nth-of-type(1)").css("border","2px solid green");
$("#m4 > span:nth-last-of-type(1)").css("border","2px solid green");
$("#m5 > span:only-child").css("border","2px solid blue");
$("#m6 > span:only-of-type").css("border","2px solid navy");
});
◆ jQuery 콘텐츠 필터 선택자
선택자 종류 | 설명 | |
---|---|---|
:contains | $("p:contains('html')") | p요소 중에서 'html'텍스트를 포함하고 있는 p요소를 선택합니다. |
:empty | $("div:empty") | div 요소 중에 자식 요소가 없는 div 요소를 선택합니다. |
:parent | $("span:parent") | span 요소 중에 자식 요소가 있는 span 요소를 선택합니다. |
:has | $("section:has(article)") | section 요소 중에서 article을 하위 요소로 가지고 있는 section 요소를 선택합니다. |
♠ jQuery 필터 선택자의 코드만 보기
$(document).ready(function() {
$("#m1 > p:contains('html')").css("border","4px solid red")
$("#m1 > p:empty").css("border","4px solid orange")
$("#m2 > span:parent").css("border","4px solid yellow")
$("#m3 > section:has(article)").css("border","4px solid green")
})
◆ jQuery 폼 필터 선택자
선택자 종류 | 설명 | |
---|---|---|
:text | $("input:text") | <input type = "text"> 요소를 선택합니다. |
:password | $("input:password") | <input type = "password"> 요소를 선택합니다. |
:imge | $("input:imge") | <input type = "imge"> 요소를 선택합니다. |
:file | $("input:file") | <input type = "file"> 요소를 선택합니다. |
:button | $("input:button") | <input type = "button">,<button> 요소를 선택합니다. |
:checkbox | $("input:checkbox") | <input type = "checkbox"> 요소를 선택합니다. |
:radio | $("input:radio") | <input type = "radio"> 요소를 선택합니다. |
:submit | $("input:submit") | <input type = "submit"> 요소를 선택합니다. |
:reset | $("input:reset") | <input type = "reset"> 요소를 선택합니다. |
:input | $(".input") | 모든 <input> 요소를 선택합니다. |
:checked | $("input:checked") | <input>요소에서 <checked>속성이 있는 요소를 선택합니다. |
:selected | $("option:selected") | <option>요소에서 <selected>속성이 있는 요소를 선택합니다. |
:focus | $("input:focus") | 현재 <input>에 포커스가 있는 요소를 선택합니다. |
:disabled | $("input:disabled") | <input>요소에서 <disabled>속성이 있는 요소를 선택합니다. |
:enabled | $("input:enabled") | <input>요소에서 <disabled>가 아닌 요소를 선택합니다. |
♠ jQuery 필터 선택자의 코드만 보기
$(document).ready(function() {
$("input:text").css("background", "red");
$("input:password").css("background", "orange");
$(":button").css("background", "yellow");
$("input:checked + label").css("background", "green");
$("option:selected").css("color", "blue");
$("textarea:disabled").css("background", "pink");
});
◆ jQuery 가시성 필터 선택자
선택자 종류 | 설명 | |
---|---|---|
:hidden | div:hidden | div요소 중 hidden인 요소를 선택합니다. |
:visible | div:visible | div요소 중 visible 요소를 선택합니다. |
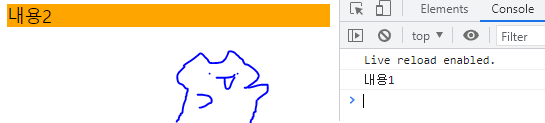
♠ jQuery 필터 선택자의 코드만 보기
$(document).ready(function() {
console.log($("div:hidden").text());
$("div:visible").css("background","orange")
});
728x90
'개념정리' 카테고리의 다른 글
jQuery 클래스 메서드 (4) | 2022.09.03 |
---|---|
jQuery 탐색 선택자 (4) | 2022.08.31 |
jQuery 속성 선택자 (3) | 2022.08.31 |
jQuery 기본 선택자 (3) | 2022.08.31 |
jQuery 개념 정리 (6) | 2022.08.29 |
댓글